有一种情况,你的商品需要带上条形码,就像你在超市买可乐会扫一下,或者带个二维码。
今天团队里的一个前端同学遇到了一个问题,就是需要根据接口拿到的数据,生成条形码并且可以点击打印出来(不是console.log的这个打印,是打印条形码的打印机打印出的纸质条码),因为应用环境是vue,所以举例用vue,因为项目的代码不能外泄,这里用npm包简单写2个一般开发够用的栗子
条形码
条形码的长相很普通,比如一般买的书后面带的那个,长相就像这样
实现起来非常简单,安装and使用
一、安装1
npm install --save jsbarcode
二、使用
vue环境1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31<template>
<svg ref="barcode" />
</template>
<script>
import JSbarcode from 'jsbarcode'
export default{
mounted() {
this._initJsBarcode();
},
methods: {
_initJsBarcode() {
let dom = this.$refs.barcode;
let options = {
width: 2, //较细处条形码的宽度
height: 100, //条形码的宽度,无高度直接设置项,由位数决定
quite: 10,
format: "CODE128",
displayValue: true, //是否在条码下方显示文字
font:"monospace",
textAlign:"center", //对齐
fontSize: 12, //字体大小
backgroundColor:"", //条码背景色
lineColor:"#000" //条码颜色
....
};
let _jsbarcode = JsBarcode(dom, "my name is hardy", options);
}
}
}
</script>
react 环境1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38import React from 'react';
import JsBarcode from 'jsbarcode';
class Barcode extends React.Component {
constructor(props) {
super(props);
}
componentDidMount() {
this._initJsBarcode();
}
_initJsBarcode(){
let _jsbarcode = JsBarcode(this.barcode, "my name is hardy", {
width: 2, //较细处条形码的宽度
height: 100, //条形码的宽度,无高度直接设置项,由位数决定
quite: 10,
format: "CODE128",
displayValue: true, //是否在条码下方显示文字
font:"monospace",
textAlign:"center", //对齐
fontSize: 12, //字体大小
backgroundColor:"", //条码背景色
lineColor:"#000" //条码颜色
....
});
}
render() {
return (
<div className="barcode-container">
<svg
ref={(ref) => {
this.barcode = ref;
}}
/>
</div>
);
}
}
export default Barcode;
html 环境1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
<html>
<head>
<meta charset="utf-8" />
<title></title>
<script type="text/javascript" src="js/jquery.min.js"></script>
<script type="text/javascript" src="js/JsBarcode.all.min.js"></script>
</head>
<body>
<svg id="svgbarcode"></svg>
<canvas id="canvascode"></canvas>
<img id="imgcode" />
<script>
$("#svgbarcode").JsBarcode('显示文案');
$("#canvascode").JsBarcode('显示文案');
$("#imgcode").JsBarcode("显示文案");
</script>
</body>
</html>
这是github上它的配置参数
github地址
二维码
二维码和条码的实现方式一样,只不过二维码用的npm包是qrcodejs2
同样的步骤
一、安装1
npm install --save qrcodejs2
二、使用1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23<template>
<div ref="qrcode"></div>
</template>
<script>
import QRCode from 'qrcodejs2'
export default{
mounted() {
this._qrcode();
},
methods: {
_qrcode(){
let _qrcode = new QRCode(this.$refs.qrcode, {
width: 132,
height: 132,
text: 'my name is hardy',
colorDark : "#000",
colorLight : "#fff",
})
}
}
}
</script>
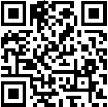
生成二维码也可以使用QRCode.js1
2
3
4
5
6
7
8
9
10<div id="qrcode"></div>
var qrcode = new QRCode("test", {
text: "https://hhardyy.com/", // 设置要生成二维码的链接
width: 128,
height: 128,
colorDark : "#000000",
colorLight : "#ffffff",
correctLevel : QRCode.CorrectLevel.H
});